Master 12-Factor App Methodology for DevOps Application Development
Learn the essentials of building scalable and maintainable cloud-native applications with the 12-Factor App methodology. This guide by Arya Aniket covers key principles like CI/CD pipelines, configuration management, and stateless processes for modern app development.
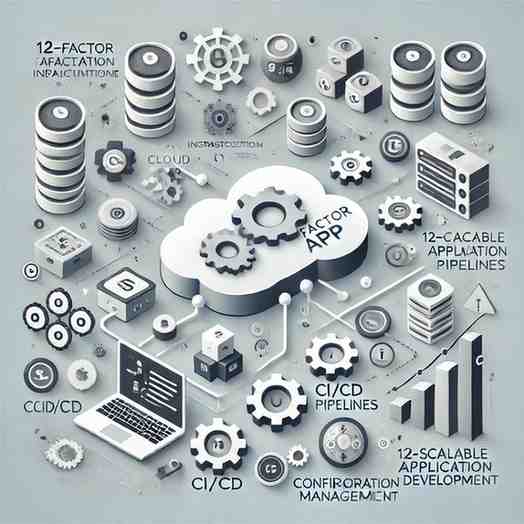
Unlock the secrets of building scalable, maintainable cloud-native applications with the 12-Factor App methodology. This course, led by expert Arya Aniket, delves into every principle of the 12-Factor App, equipping you with the skills to design resilient applications using best practices. Learn how to utilize modern tools and CI/CD pipelines, efficiently manage configurations, and develop applications that thrive in a fast-evolving software landscape.
In today's fast-paced software development world, creating scalable and easily maintainable applications is essential for long-term success. The 12-Factor App methodology lays out a set of best practices for building cloud-native applications that are robust, easy to scale, and straightforward to maintain. This course introduces the fundamental principles of 12-Factor Apps, guiding you through each aspect and demonstrating how to implement them in your projects. You will learn how to design applications that are simple to deploy and manage, use externalized configuration management for environment-specific settings, and build stateless, horizontally scalable applications. Additionally, you’ll explore tools and techniques such as continuous integration and continuous deployment (CI/CD) pipelines, which streamline the development and deployment of modern applications. By the end of the course, you'll possess the expertise to apply the 12-Factor App methodology, creating resilient, cloud-native applications that meet today’s demands.
- Codebase
- Dependencies
- Concurrency
- Processes
- Backing Services
- Config
- Build, Release, and Run
- Port Binding
- Disposability
- Dev-Prod Parity
- Logs
- Admin Processes
Codebase
A codebase refers to the complete collection of source code used to build a particular software application or system. It encompasses all the files, libraries, and resources needed to develop, maintain, and deploy the software. Here’s a simple breakdown:
- Components of a Codebase:
- Source Code Files: The actual programming files written in languages like Python, Java, JavaScript, etc.
- Configuration Files: Files that specify settings for the application (e.g., database connections, API keys).
- Documentation: Guides and comments within the code that explain how to use or understand the software.
- Dependencies: External libraries or packages that the code relies on to function properly.
- Purpose:
- Development: The codebase is where developers write and test new features or fix bugs.
- Version Control: It is often managed with version control systems (like Git) to track changes, collaborate with others, and manage different versions of the software.
- Organization:
- A well-organized codebase is essential for maintainability, readability, and ease of collaboration among team members. This often involves using consistent naming conventions, directory structures, and documentation practices.
In summary, the codebase is the foundation of a software project, containing everything necessary to create, run, and manage the application.
- Collaboration.
- Git.
- Remote Repository.
- Github, Bitbucket, Gitlab
Dependencies
Dependencies refer to external libraries, frameworks, or components that a software application requires to function properly. These are pieces of code written by others that provide specific functionalities or features, allowing developers to avoid reinventing the wheel. Here’s a simple overview:
- Types of Dependencies:
- Library Dependencies: Code libraries that offer reusable functions or classes, such as jQuery for JavaScript or NumPy for Python.
- Framework Dependencies: Larger structures that provide a foundation for building applications, like React for frontend development or Django for backend development.
- Runtime Dependencies: Software components needed for the application to run, like database drivers or web servers.
- Purpose:
- Functionality: Dependencies provide pre-built solutions for common problems, allowing developers to focus on building their application's unique features.
- Efficiency: Using established libraries or frameworks can speed up development and reduce the likelihood of bugs, as they are often well-tested and maintained by the community.
- Managing Dependencies:
- Package Managers: Tools like npm (for JavaScript), pip (for Python), or Maven (for Java) are used to manage dependencies, making it easy to install, update, and remove them.
- Version Control: It's important to track the versions of dependencies to avoid compatibility issues, especially when different libraries might depend on specific versions of other libraries.
In summary, dependencies are crucial components that enhance the functionality of applications by allowing developers to leverage existing code, promoting faster and more efficient software development.
- Isolate
- Explicitly declare and Isolate Dependencies
Example: Python => requirement.txt, Node => package.json, package-lock.json, GoLang => go.mod
Concurrency
Concurrency in the context of the 12-Factor App methodology refers to the ability of an application to handle multiple processes or requests simultaneously. This principle is essential for building scalable and efficient applications, especially in cloud environments. Here’s a simple overview:
Key Aspects of Concurrency in 12-Factor Applications:
- Scaling Out:
- The application should be designed to run multiple instances of processes or services concurrently. This allows it to handle increased load by distributing requests across these instances, often referred to as "horizontal scaling."
- Stateless Processes:
- Each instance of the application should be stateless, meaning that it does not rely on any specific data stored in memory. Instead, any necessary data should be stored externally (like in a database or cache). This approach allows for easy scaling since any instance can handle any request without needing to access previous state information.
- Concurrency Models:
- Applications can implement different concurrency models, such as:
- Thread-based concurrency: Using threads within a process to handle multiple tasks simultaneously.
- Event-driven concurrency: Utilizing events and callbacks to manage asynchronous tasks.
- Process-based concurrency: Running multiple processes to manage separate tasks or requests.
- Applications can implement different concurrency models, such as:
- Worker Processes:
- For background jobs or long-running tasks, worker processes can be employed. These are separate processes that handle specific jobs and can run concurrently with the main application processes.
- Load Balancing:
- To effectively manage concurrent requests, load balancers can be used to distribute incoming traffic evenly across multiple application instances, ensuring no single instance becomes overwhelmed.
Processes
Key Aspects of Processes in 12-Factor Applications:
- Statelessness:
- Each process should be stateless, meaning it does not retain any information between requests. Any necessary state or data should be stored externally (e.g., in a database or cache). This allows processes to be easily replicated or scaled without issues.
- Concurrency:
- Multiple processes can run concurrently to handle different requests or tasks. This concurrency allows the application to be more responsive and to manage higher loads effectively.
- Process Types:
- Web Processes: Handle incoming HTTP requests from users. These processes typically run behind a web server and are responsible for serving web pages or APIs.
- Worker Processes: Perform background tasks, such as processing jobs, sending emails, or performing scheduled tasks. These are often decoupled from the web processes and can be scaled independently.
- Management Processes: Execute administrative tasks, such as database migrations, data seeding, or maintenance scripts. These are usually run manually or as part of a deployment pipeline.
- Scaling:
- The application can scale by running multiple instances of its processes. For example, if an application experiences increased traffic, additional web processes can be spun up to handle the load. This is often referred to as horizontal scaling.
- Portability:
- Processes should be designed to run in isolation, meaning they can be deployed and executed in different environments (development, staging, production) without modification.
Benefits of Managing Processes:
- Resilience: If one process fails, others can continue to function, ensuring the application remains available.
- Efficiency: Allows for better resource utilization, as different types of processes can be optimized for specific tasks.
- Maintainability: Clear separation of concerns makes it easier to manage and update different parts of the application.
In summary, processes in 12-Factor Apps are essential units of execution that allow applications to handle requests efficiently and scale effectively while maintaining a clear and manageable architecture.
Example:
- Visit Count
- Session Info
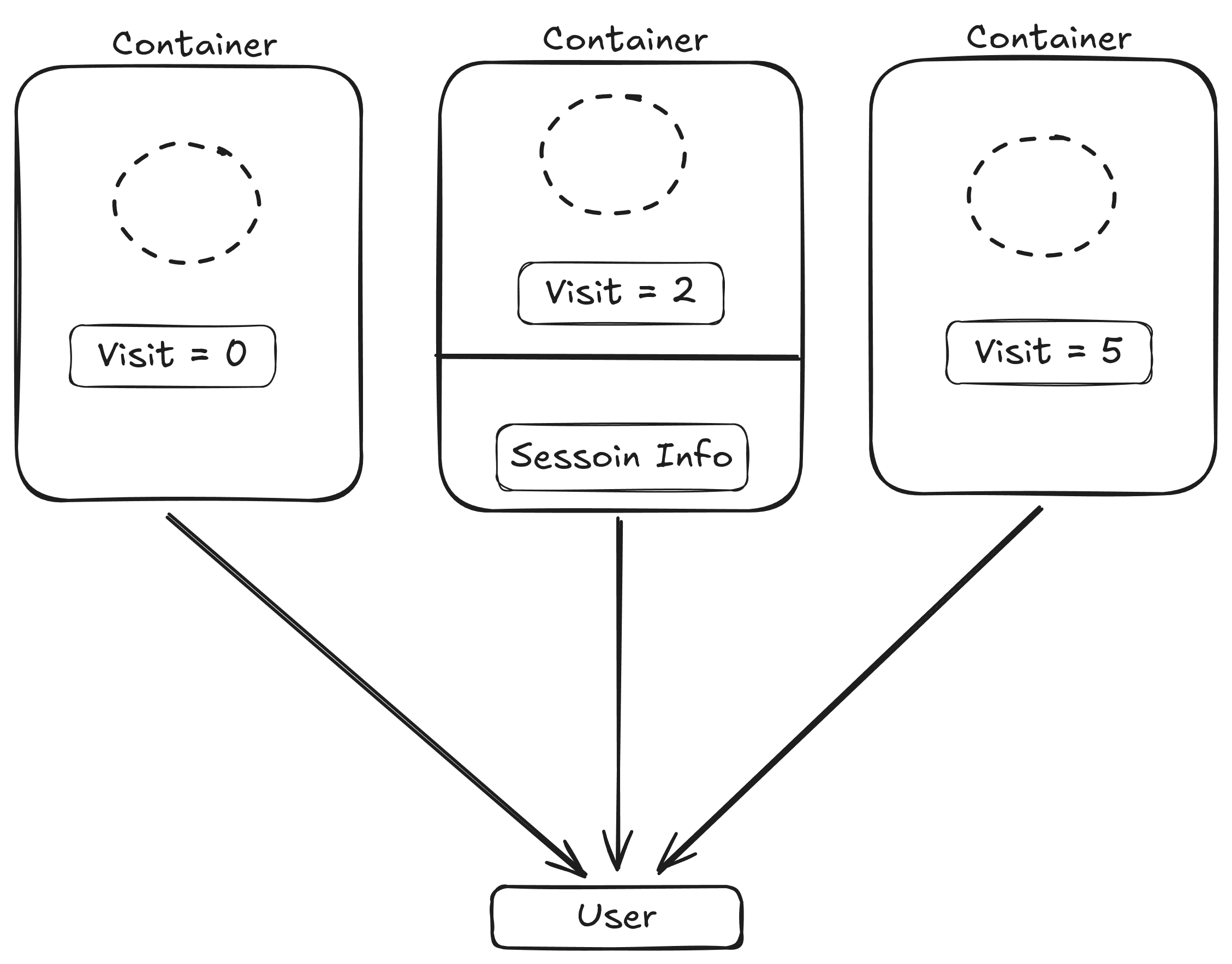
- Twelve-factor processes are stateless and shaving-nothing
- Sticky sessions are a violation of the twelve-factor and should never be used or relied upon
- That's why we should store the session-like thing in the backing session
- Redis, Memcache etc.
Backing Services
A backing service is a service that an application relies on its functionality.
Backing Services refer to external resources or services that a software application depends on to function properly. These can include databases, message queues, caching systems, and other services that support the application’s operations. Here’s a simple breakdown of what backing services are and their significance in the 12-Factor App methodology:
Key Aspects of Backing Services:
- Externalized Dependencies:
- Backing services are treated as external dependencies, meaning they should not be bundled with the application code. Instead, they are accessed over a network (e.g., HTTP, TCP).
- Types of Backing Services:
- Databases: Such as PostgreSQL, MySQL, or MongoDB, used to store application data.
- Message Queues: Like RabbitMQ or Kafka, used for asynchronous communication between different parts of an application.
- Caching Systems: Such as Redis or Memcached, used to store frequently accessed data for quick retrieval.
- File Storage: Services like Amazon S3 or Google Cloud Storage for storing and retrieving files.
- Third-party APIs: External services that provide functionalities like payment processing, email delivery, or authentication.
- Management:
- Backing services should be easily configurable through environment variables, allowing developers to switch between different services (like development and production databases) without changing the application code.
- Service Discovery:
- Applications should be able to discover and connect to these backing services dynamically, ensuring flexibility and reducing hardcoding of service locations or credentials.
Benefits of Using Backing Services:
- Separation of Concerns: By externalizing dependencies, applications can focus on their core functionalities while relying on specialized services for other tasks.
- Scalability: Backing services can be scaled independently from the application, allowing for more efficient resource management.
- Flexibility: It becomes easier to replace or upgrade backing services without affecting the core application logic.
Conclusion:
In summary, backing services are essential components that support the functionality of an application while allowing it to remain flexible, maintainable, and scalable. By treating these services as external dependencies, developers can create more modular and robust applications that can easily adapt to changing requirements and environments.
Example: Databases, such as Mysql, Postgres, SMTP, Caching System.
Config
Config refers to the configuration settings and parameters that an application uses to operate. In the context of the 12-Factor App methodology, managing configuration effectively is crucial for building scalable and maintainable applications. Here’s a simple overview of what config entails:
Key Aspects of Config:
- Separation from Code:
- Configuration settings should be stored outside of the application code. This allows you to change settings (like database connections or API keys) without modifying the codebase itself, promoting flexibility and reducing deployment risks.
- Environment-Specific Settings:
- Configurations often vary between different environments (development, testing, production). For example, you might use a local database in development and a cloud database in production. By externalizing config, you can easily switch between these environments without changing the code.
- Storage Methods:
- Configuration settings can be stored in various ways:
- Environment Variables: Commonly used for storing sensitive information like API keys or database passwords.
- Configuration Files: Files (like JSON, YAML, or INI) that contain settings, which can be loaded by the application at runtime.
- Service Discovery: In cloud-native applications, config can be retrieved from centralized configuration services or secret management tools (e.g., AWS Secrets Manager, HashiCorp Vault).
- Configuration settings can be stored in various ways:
- Dynamic Configuration:
- The application should be able to read configuration settings at runtime, allowing changes to take effect without restarting the application. This can be achieved by using environment variables or configuration management tools.
Benefits of Managing Config:
- Flexibility: Changes to configuration can be made without deploying new code, allowing for quicker responses to changing requirements.
- Security: Sensitive information can be managed securely, reducing the risk of exposing credentials in the codebase.
- Environment Management: Simplifies the process of moving the application between different environments, ensuring that the correct settings are used in each context.
Conclusion:
In summary, effective configuration management is essential for the 12-Factor App methodology. By keeping config settings separate from the code and making them easily adjustable based on the environment, developers can create applications that are more flexible, secure, and easier to maintain.
- Environment Variables .env.
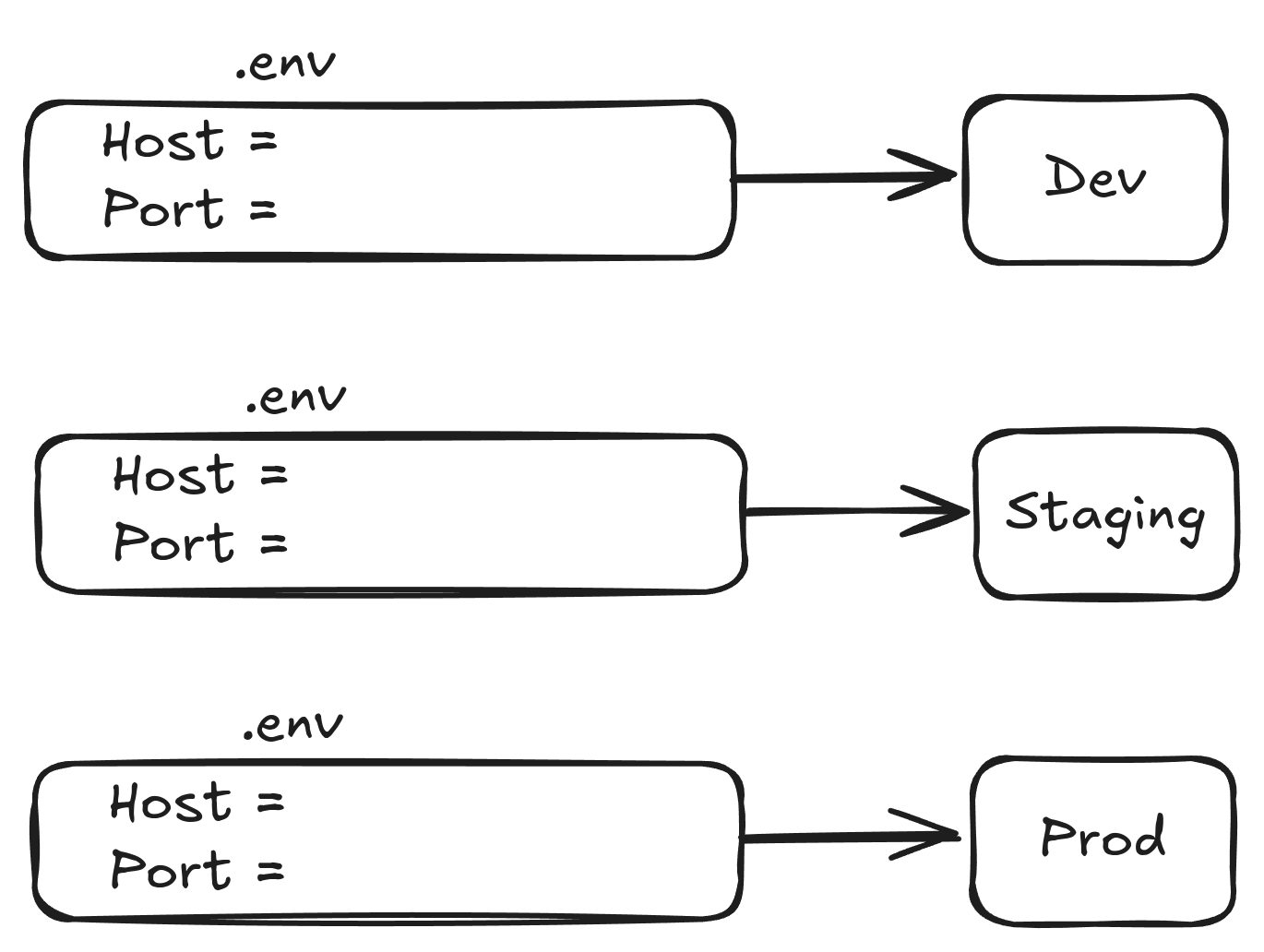
Build, Release, and Run
Build, Release, and Run is a key principle in the 12-Factor App methodology that emphasizes the separation of the application lifecycle into distinct stages. This approach enhances the deployment process, promotes consistency, and improves maintainability. Here’s a breakdown of each stage:
1. Build
- Definition: The build stage involves compiling and assembling the application code along with its dependencies into a deployable artifact.
- Process:
- Source code is fetched from a version control system (e.g., Git).
- Dependencies are resolved and packaged together.
- The application is compiled (if applicable) into a runnable form (e.g., a binary, Docker image, or jar file).
- Key Considerations:
- The build process should be automated using Continuous Integration (CI) tools, ensuring that every change to the codebase triggers a build and that it consistently produces the same output.
2. Release
- Definition: The release stage involves taking the built artifact and combining it with the configuration settings specific to the deployment environment (e.g., production, staging).
- Process:
- The built artifact is pushed to a repository or deployment environment.
- Environment-specific configurations (such as environment variables) are applied.
- The release is versioned, allowing for easy rollbacks and tracking of changes.
- Key Considerations:
- The release process should be automated using Continuous Deployment (CD) tools to ensure that releases are consistent and can be deployed reliably.
- This stage should produce a release that is ready for deployment, separate from the build artifact.
3. Run
- Definition: The run stage involves executing the release in the production environment and ensuring it operates correctly.
- Process:
- The released application is started in a production environment (e.g., on a server or in a cloud service).
- The application responds to user requests and performs its intended functions.
- Key Considerations:
- The run environment should be identical to the release environment to minimize discrepancies.
- Monitoring and logging should be implemented to track application performance, detect issues, and gather insights for future improvements.
- The run stage should allow for graceful shutdowns and restarts to minimize downtime and impact on users.
Summary
The Build, Release, and Run principle of the 12-Factor App methodology encourages a clear separation between these stages of the application lifecycle. This separation leads to more predictable, automated, and repeatable processes, ultimately resulting in higher-quality software that is easier to manage and deploy. By following this principle, teams can ensure smoother deployments, quicker feedback loops, and better collaboration between development and operations.
- The twelve-factor app uses strict separation between the build, release, and run stages.
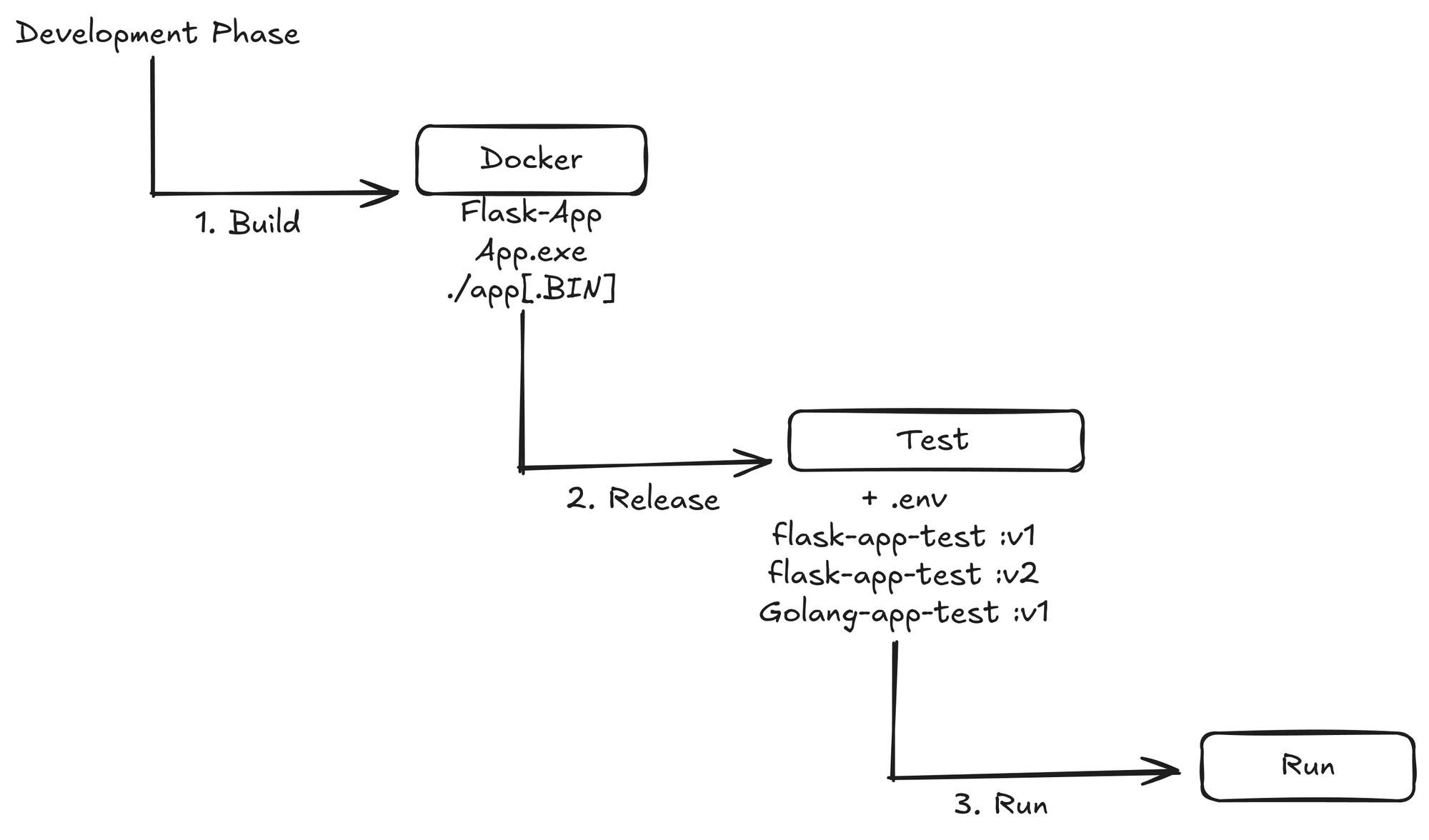
Port Binding
Port Binding is a principle in the 12-Factor App methodology that emphasizes how applications should be self-contained and expose services through a specified port. Here’s a simple breakdown of what port binding means and its significance:
Key Aspects of Port Binding:
- Self-Containment:
- An application should be capable of running as a standalone service without relying on a web server or other external components. This means it should listen for incoming requests directly on a specified port.
- Service Exposure:
- By binding to a port, the application exposes its functionality over the network. For example, a web application might listen on port 80 (HTTP) or 443 (HTTPS), while a REST API might listen on a different port, like 3000 or 8080.
- No Pre-Configured Web Server:
- Unlike traditional applications that may depend on an external web server (like Apache or Nginx), 12-Factor Apps include the web server as part of the application. This reduces complexity and makes deployment simpler.
- Environment Independence:
- Since the application binds to a port, it can be easily deployed in different environments (development, staging, production) without needing to modify the codebase. The specific port can be defined in configuration settings or environment variables.
Benefits of Port Binding:
- Simplicity: Eliminates the need for external server configuration, making the application easier to deploy and manage.
- Scalability: Multiple instances of the application can be run simultaneously on different ports, allowing for horizontal scaling.
- Consistency: Ensures that the application behaves the same way regardless of where it is deployed, as it handles incoming requests directly.
Summary:
In summary, Port Binding in the 12-Factor App methodology encourages applications to be self-contained services that listen for incoming requests on a specified port. This principle promotes simplicity, scalability, and consistency, making it easier to develop, deploy, and manage modern applications in cloud environments.
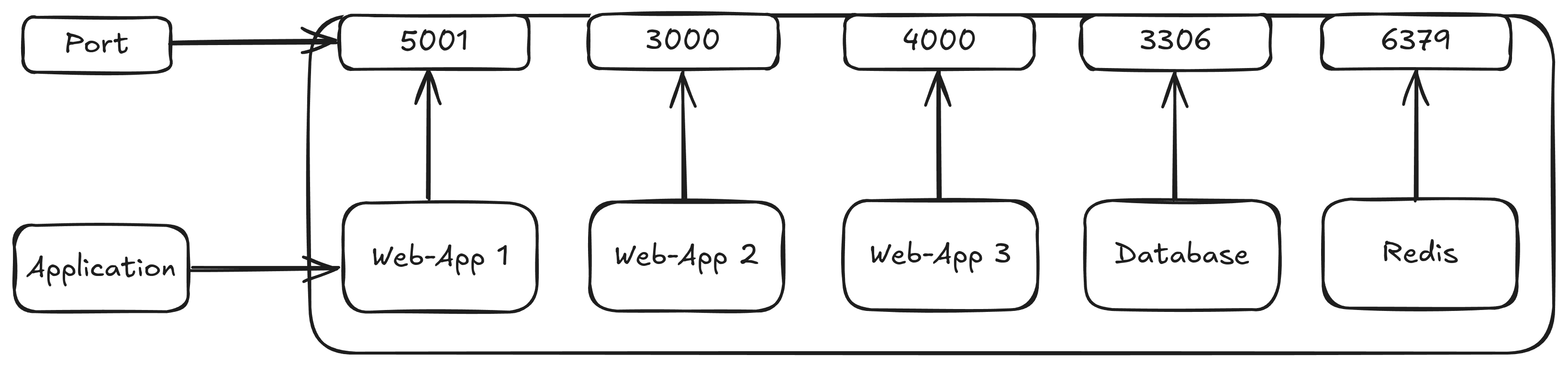
Disposability
Disposability is a key principle in the 12-Factor App methodology that emphasizes the ability of an application to start up and shut down quickly and gracefully. This principle is crucial for building resilient and scalable cloud-native applications. Here’s a simple overview of what disposability means and its importance:
Key Aspects of Disposability:
- Rapid Startup and Shutdown:
- Applications should be able to start up quickly and shut down gracefully. This enables efficient scaling, deployment, and maintenance.
- Graceful Termination:
- When a process receives a shutdown signal (such as during scaling down or updating), it should have the ability to complete any ongoing requests and clean up resources (like database connections) before terminating. This ensures a smooth transition and avoids data loss.
- Statelessness:
- By designing applications to be stateless (where no client-specific information is retained), any instance can handle any request. This allows for quick replacement of processes without affecting the overall application functionality.
- Health Checks:
- Implementing health checks can help determine if an application instance is running properly. If an instance fails a health check, it can be automatically replaced with a new instance, enhancing reliability.
Benefits of Disposability:
- Scalability: Disposability allows applications to scale up and down quickly in response to changes in demand, making them more responsive to user needs.
- Resilience: If an application instance fails, it can be quickly replaced without causing significant downtime or disruption.
- Easier Maintenance: Updates or maintenance can be performed more seamlessly, as new instances can be spun up and old ones gracefully shut down.
Summary:
In summary, Disposability in the 12-Factor App methodology promotes the design of applications that can start and stop quickly and handle shutdowns gracefully. This principle enhances the scalability, resilience, and maintainability of cloud-native applications, allowing them to adapt to changing demands and maintain high availability.
Dev-Prod Parity
Dev/Prod Parity in the 12-Factor App is about keeping your development and production environments as similar as possible. The idea is that what you build and test during development should behave the same way in production.
- Reduce Time Gaps: The code, configuration, and deployment processes should stay up to date across development, testing, and production environments. Deploy frequently to reduce the gap between writing code and running it in production.
- Minimize Environmental Differences: Keep development, staging, and production environments as similar as possible. This includes using the same dependencies, operating system versions, and environment variables.
- Streamline Deployment: Automate and standardize deployment processes to ensure that what works in the development and testing phases works seamlessly in production.
Logs
Logs are records that capture events, actions, or changes occurring in a software application or system. They are essential for monitoring, debugging, and analyzing the performance and behavior of applications. Here’s a simple breakdown:
- What They Contain: Logs typically include details like timestamps, event types (errors, warnings, information), and messages that describe what happened (e.g., a user logged in, an error occurred, or a file was accessed).
- Types of Logs:
- Application Logs: Record activities within the application itself, such as user actions or background processes.
- System Logs: Capture information about the operating system and hardware, such as system errors or resource usage.
- Access Logs: Track requests made to a server, including IP addresses, request types, and response statuses.
- Purpose:
- Debugging: Helps developers identify and troubleshoot issues in the application.
- Monitoring: Provides insights into application performance and user behavior.
- Auditing: Maintains a record of actions for security and compliance purposes.
In summary, logs are valuable tools for understanding what’s happening in your application or system, helping you ensure everything runs smoothly and troubleshoot issues when they arise.
Admin Processes
Admin Processes refer to tasks and activities that are typically carried out by system administrators or operations teams to manage and maintain software applications, systems, or infrastructure. These processes ensure that applications run smoothly and securely. Here’s a simple overview:
- What They Include:
- Monitoring: Keeping an eye on system performance, resource usage, and application health.
- Scaling: Adjusting resources (like adding servers) to handle increased traffic or workload.
- Backup and Recovery: Creating copies of data to prevent loss and ensuring systems can be restored in case of failure.
- Updates and Maintenance: Installing software updates, patches, and performing routine maintenance tasks to keep systems secure and efficient.
- Purpose:
- Stability: Ensuring that applications remain reliable and available to users.
- Security: Protecting systems from vulnerabilities and unauthorized access.
- Performance Optimization: Improving system efficiency and responsiveness.
- Characteristics:
- Automatable: Many admin processes can be automated using scripts or tools, reducing manual effort and the chance of human error.
- Stateless: Admin processes are usually independent of the application state, meaning they can run without affecting ongoing user interactions.
In essence, admin processes are crucial for the effective operation and management of software applications and systems, helping to maintain their health and performance.